Imagine building a beautiful application, only to discover it crashing and misbehaving in the hands of users. Frustrating, isn’t it? This is where debugging comes into play. Debugging is the systematic process of identifying and fixing issues in your code. It ensures that your software works as intended, minimizing errors and improving user experience.
Understanding the Problem
Before diving into the debugging process, take a step back and make sure you understand the problem at hand. Reproducing the issue and identifying its scope are essential steps.
When faced with a bug, start by reading error messages and studying user reports. Often, these messages provide valuable clues about what went wrong. If possible, try to reproduce the problem in a controlled environment. Understanding the circumstances under which the bug occurs will help you narrow down the cause.
Leveraging Debugging Tools
Debugging tools are a developer’s best friend. They provide invaluable assistance in identifying and resolving issues efficiently. Let’s explore some popular debugging tools:
Integrated Development Environments (IDEs)
Modern IDEs offer built-in debuggers that allow you to step through your code, set breakpoints, and examine variables. Visual Studio Code, PyCharm, and Xcode are examples of IDEs with powerful debugging capabilities. Familiarize yourself with the debugger of your chosen IDE and leverage its features to your advantage.
Command-Line Debuggers
Command-line debuggers like GDB (GNU Debugger) are particularly useful when working with lower-level languages such as C or C++. They allow you to track the execution flow, set breakpoints, and examine memory.
Profilers and Performance Analyzers
Performance issues can be tricky to diagnose without specialized tools. Profilers and performance analyzers help you identify bottlenecks and optimize your code. Xcode Instruments, for instance, provides deep insights into the performance of iOS applications.
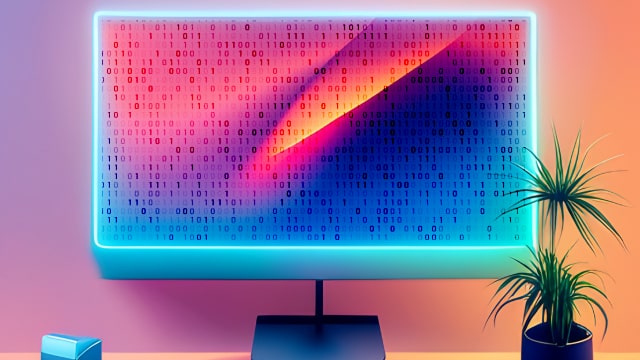
Analyzing Error Logs
Error logs are a goldmine of information when it comes to debugging. They provide a detailed account of what went wrong and where. Analyzing error logs effectively can save you hours of frustration. Here are some tips:
- Look for specific error codes or messages that indicate the root cause of the issue.
- Pay attention to timestamps and the sequence of events leading to the error.
- Scan the log for patterns or recurring errors that might provide additional context.
- Use logging frameworks or libraries to generate informative and structured logs.
Printing Relevant Variables
Sometimes, the best way to understand what’s happening in your code is to get up close and personal with the variables. Printing relevant variables at critical points can help you uncover hidden bugs. Consider the following techniques:
- Strategic Printing: Identify crucial variables and print their values at critical junctures in your code. This allows you to track their changes and identify discrepancies.
- Conditional Printing: Use conditional statements to print variables only when specific conditions are met. This approach can help you narrow down problematic sections of code.
Removing Unnecessary Code
Unnecessary code is like clutter in your program. It not only makes your code harder to read and maintain but also increases the chances of introducing bugs. During debugging, take the opportunity to clean up your codebase. Here’s how:
- Unused Variables and Functions: Scan your code for variables and functions that are not used. Removing them simplifies your codebase and reduces the potential for confusion.
- Simplify Loops and Conditions: Review loops and conditions to ensure they are necessary. Simplifying complex logic can make your code more readable and less error-prone.
- Refactor and Optimize: As you debug, identify areas for improvement. Refactor and optimize your code to make it cleaner, more efficient, and easier to debug in the future.
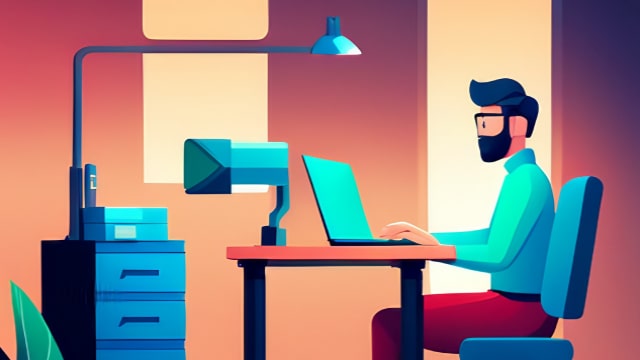
Testing Incrementally
Debugging large chunks of code all at once can be overwhelming. Instead, break down complex problems into smaller, manageable pieces. Incremental testing allows you to focus on one area at a time and catch bugs early on. Consider the following techniques:
- Unit Testing and Test-Driven Development (TDD): Write unit tests to verify individual components of your code. TDD encourages you to write tests before implementing the code itself, promoting a more reliable and debug-friendly development process.
- Step-by-Step Execution: Debugging can be more effective when you execute your code step by step. Observe the changes in variables and data structures as you progress through each line. This approach helps pinpoint the exact location of bugs.
Conclusion:
Debugging is a skill that evolves with experience and practice. By following these essential coding tips, you’ll be well-equipped to tackle bugs like a pro. Remember to understand the problem, leverage debugging tools, analyze error logs, print relevant variables strategically, remove unnecessary code, and test incrementally.
Mastering the art of debugging will make you a more confident and efficient developer, enabling you to deliver reliable and bug-free software.
Now, go forth, debuggers, and tame those pesky bugs!